Python (2): List & Set
- Harmony Pang
- Aug 8, 2023
- 1 min read
Updated: Sep 14, 2023
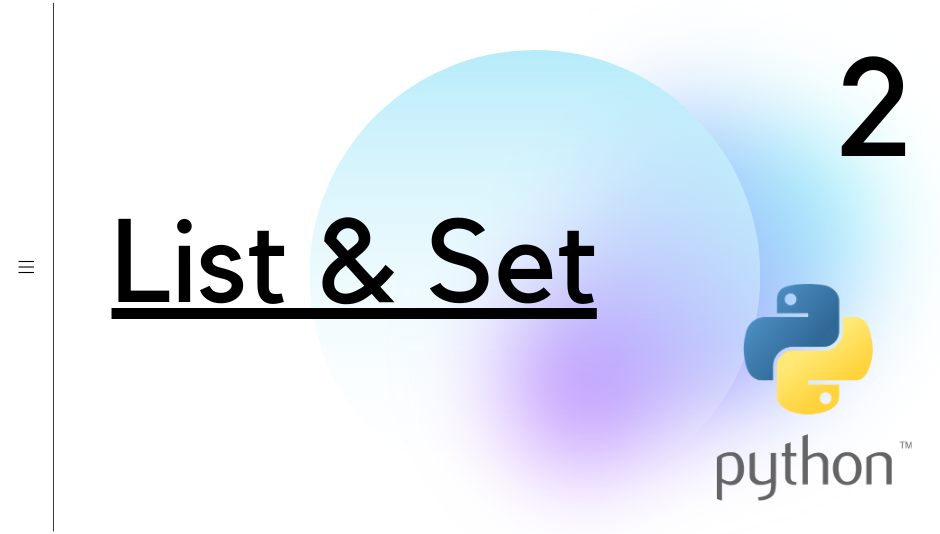
This time we'll talk about an effective combination of 3 functions in Python: [:], sorted(), set().
[:]
It is usually used on a list. Assume there's a list a.
a[:] allows you to replace the original list a with a new list.
sorted()
It sorts almost all data types in ascending order (e.g. list, tuple, set, dictionary...).
You can sort in descending order by adding parameter reverse=True, which is sorted(a, reverse=True).
set()
It turns a list into a set.
It automatically remove all the duplicates in the list.
However, the order of the elements in the list may be unordered.
Let's see an example:
a[:] = sorted(set(a))
return a
Assume a is a list contains [2, 3, 3 ,4, 4, 5, 5, 6, 6].
set() first turns the list into a set and remove its all duplicates.
However, set() will make the set unordered, so the set now may be {2, 4, 3, 6,5}.
sorted() then sort the set in ascending order and turn back it into a list again, so the list now is [2, 3, 4, 5, 6].
The sorted set now assigns to a[:], which allows the sorted list to replace the original list.
Return a, a now is [2, 3, 4, 5, 6].
Congratulations on completing this tutorial!
You now have the knowledge and tools to excel in this skill.
See You in Python (3)!
© 2023 Harmony Pang. All rights reserved.
Comments