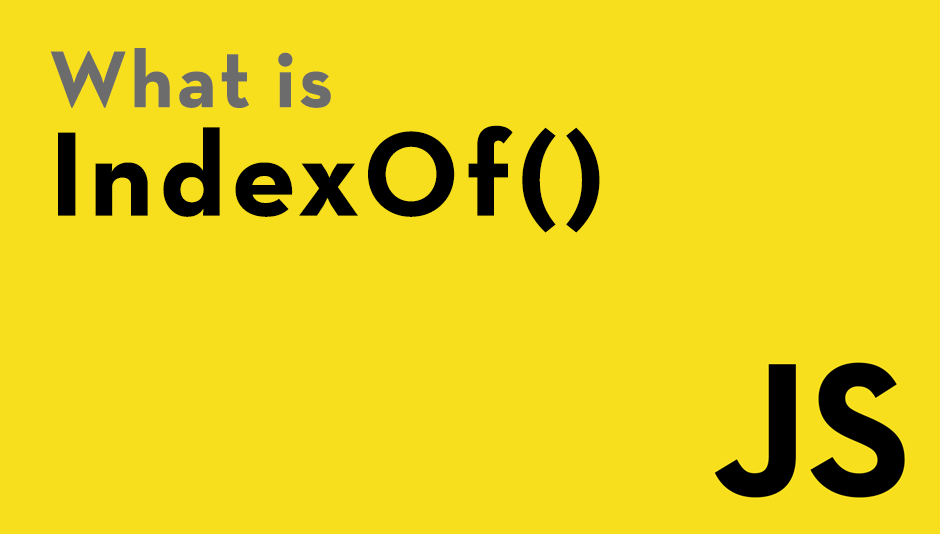
indexOf() is a JavaScript method finding the first occurance of the value in the parenthesis() in a string or array.
It may sound weird at first but let's see the following example:
a.indexOf(b)
It's just like asking "Hey, may I know where is b first found in a?".
That means, a is a string or array, b is the the value that we're going to search for in a.
And, if b is really found in a, then it'll return the index where b is first found.
For example, if a is "flower", b is "fl", then it returns 0 because "fl" is first found at the first character of "flower", which is "f", the index[0] character.
The return value of indexOf() can be 0, 1, 2, 3... or -1.
If it's a positive integer, it indicates the index of the first occurance of b in a.
However, if it's -1, that means b cannot found anywhere in a.
Let's see more advanced usage of indexOf().
Case Study:
if (!strs.length) {
return "";
}
let prefix = strs[0];
for ( i = 1; i < strs.length; i++) {
while (strs[i].indexOf(prefix) !== 0) {
prefix = prefix.slice(0, -1);
if (prefix == 0) {
return "";
}
}
}
return prefix;
In this case, the program is trying to find the longest common prefix in the string array "strs".
Let's say strs contains 2 strings, "flower" and "flow", which "flower"= strs[0], "flow" = strs[1].
If we only focus on the line using indexOf(), which is "(strs[i].indexOf(prefix) !== 0)", we can see that a now is str[i], b now is prefix.
"strs[i].indexOf(prefix)" means "May I know where is prefix first found in strs[i]?".
Let's say i = 1, then strs[i] = strs[1], strs[1] = "flow".
Now, according to the line above "prefix = strs[0]", we know that prefix = "flower".
So, "strs[i].indexOf(prefix)" is asking "Where is "flower" first found in "flow"?
Then, of course, as "flower" cannot be found anywhere in "flow", it'll return -1.
The part worth mentioning is, when indexOf() is used in denial (!==), it represents the opposite case of the number after !==.
In our example, "!==0" means the opposite of 0.
As we know that 0 means prefix is first found at the first character of str[i], !==0 now means the opposite, which means prefix is not found at the first character of str[i].
Contrarily, if !== -1, that means prefix is found (anywhere) in str[i], without restricting where it's found.
Congratulations on completing this tutorial!
I hope you found it helpful and informative.
Don't be afraid to experiment and try out these skills on your own.
The more you practice, the more you'll improve.
© 2023 Harmony Pang. All rights reserved.
Comments