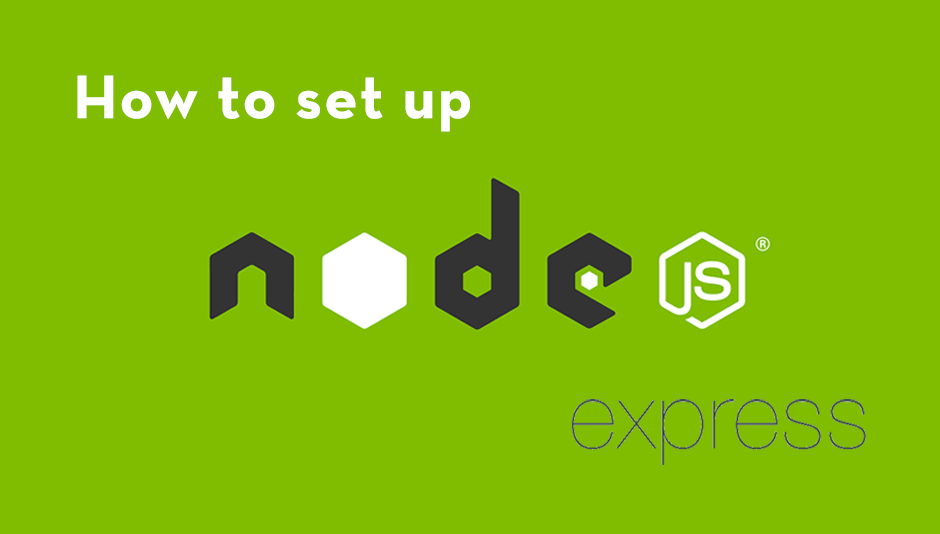
In this article, we'll go through how to set up Node.js and Express.js in Visual Studio Code with best practices.
1. Install Node.js in your local computer
To use Node.js for development, it needs to be pre-installed in your local computer.
The node package manager (npm) is included in the installation of Node.js.
You can then use npm to install Express.js, the Node.js web application framework.
Visit the official Node.js website: https://nodejs.org
Download and install the LTS version of Node.js
2. Initialize a new Node.js project
To apply Node.js in your project, it has to be initialized.
It creates a new Node.js project and a package.json file.
The package.json file is auto-generated after initializing Node.js app, which stores the dependencies and information of your app for npm to run.
Dependencies are libraries, moudules, packages (e.g. node.js, express.js, react.js…).
Creat a new folder in file manager, right-click and select "Open terminal", type the following command and the folder will be opened in VS Code:
code .
Open the terminal in VS Code, type the following command. It initializes a new Node.js project and create a package.json file for your app.
npm init
3. Create index.js file as a starting point of your app
If you click into the package.json file, you'll see "main": "index.js", which means the app set the index.js file as the default main file of the app.
Therefore, you need to create an index.js file to be the entry of your app.
Create and name a new file "index.js" in your project folder.
4. Install Express.js using npm
Now is the time to install Express.js.
As I mentioned before, Express.js is a Node.js package, so it does not need to be pre-installed in your local computer but using npm to install.
Type the following command in the terminal. It installs Express.js package in your Node.js project and save Express.js as a dependency in the package.json file.
npm install express --save
5. Set up a Express server
In index.js file, type:
const express = require("express")
const app = express();
app.use(express.urlencoded({extended:false}));
app.listen(3000);
Explanation:
const express = require("express")
▲ require() is a Node.js function to import modules. "express" is the module to be imported. It then import into the constant variable express to make sure it not to be modified.
const app = express();
▲express() create a new Express.js instance. It then assign into constant variable app to make sure only this single Express.js instance is used throughout the application.
app.use(express.urlencoded({extended:false}));
▲ app.use() call the middleware package in Node.js to handle HTTP requests. express.urlencoded() call the built in middleware function urlencoded() to encode the HTTP requests. {extended:false} means using built-in library to encode the HTTP requests instead of third-party library.
app.listen(3000);
▲ app.listen() set the app server to the port 3000. When you use web browser to navigate to http://localhost:3000, you are sending a HTTP request to your app server. The app server will then send back a response to your browser and the content will be displayed on your screen.
That's it! You're now sucessfully set up a server using Node.js and Express.js for your app.
I hope you found it valuable and gained a good understanding of the topic.
Remember, practice is key to mastering these skills, so don't hesitate to experiment and apply what you've learned on your own.
© 2023 Harmony Pang. All rights reserved.
Comments